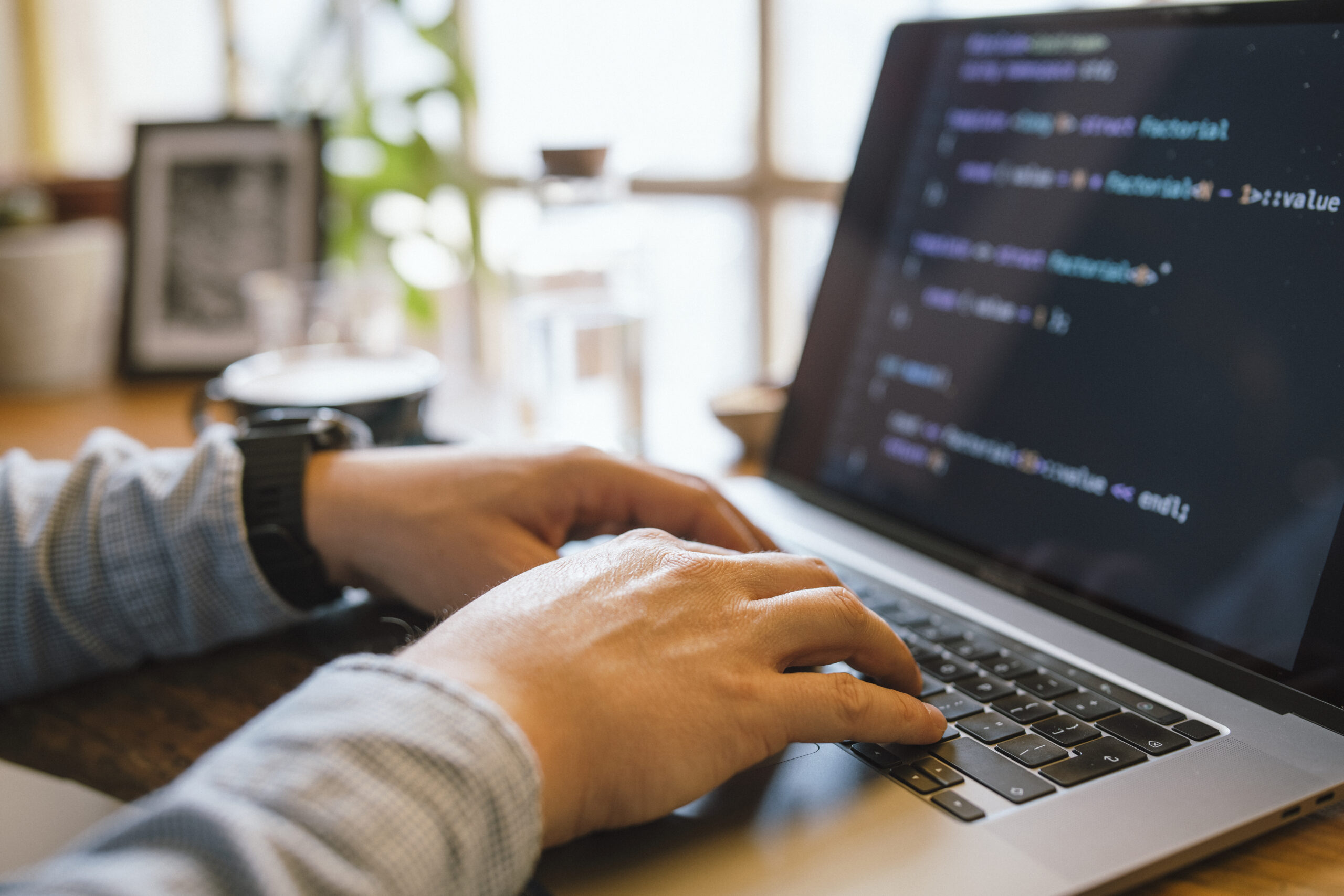
Debugging is The most critical — but typically forgotten — abilities in the developer’s toolkit. It isn't really just about fixing broken code; it’s about comprehension how and why points go wrong, and Studying to Feel methodically to resolve troubles successfully. Irrespective of whether you are a beginner or a seasoned developer, sharpening your debugging techniques can save hrs of disappointment and considerably help your productivity. Listed here are a number of methods to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
Among the quickest methods developers can elevate their debugging competencies is by mastering the applications they use on a daily basis. Even though composing code is a single A part of development, recognizing tips on how to communicate with it successfully through execution is Similarly critical. Modern day development environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these equipment can do.
Acquire, by way of example, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code over the fly. When utilised properly, they Enable you to observe particularly how your code behaves in the course of execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-close developers. They assist you to inspect the DOM, check community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can transform irritating UI difficulties into manageable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management around working procedures and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation control techniques like Git to be aware of code record, find the exact second bugs have been launched, and isolate problematic modifications.
Eventually, mastering your instruments suggests likely further than default settings and shortcuts — it’s about developing an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not shed at the hours of darkness. The greater you know your tools, the more time you can spend resolving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more significant — and infrequently neglected — methods in powerful debugging is reproducing the trouble. Just before jumping into your code or earning guesses, builders want to create a consistent environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What steps led to The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise problems under which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If The problem seems intermittently, think about producing automatic exams that replicate the sting cases or condition transitions included. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, the issue could be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than specific configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and converse far more Plainly using your staff or people. It turns an summary grievance into a concrete challenge — and that’s in which developers thrive.
Browse and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Improper. As opposed to seeing them as irritating interruptions, builders really should study to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, wherever it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Start off by studying the information thoroughly and in full. Lots of developers, especially when underneath time stress, look at the primary line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These issues can manual your investigation and place you toward the dependable code.
It’s also helpful to grasp the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can drastically accelerate your debugging procedure.
Some glitches are imprecise or generic, and in People conditions, it’s essential to examine the context where the mistake occurred. Check out similar log entries, enter values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger issues and provide hints about prospective bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A great logging technique starts with knowing what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic information during enhancement, Facts for normal functions (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine troubles, and FATAL when the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Target important events, condition alterations, input/output values, and important selection points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently discover and deal with bugs, developers need to solution the process like a detective fixing a thriller. This way of thinking allows stop working complicated concerns into workable pieces and follow clues logically to uncover the root trigger.
Start off by gathering evidence. Look at the indicators of the situation: mistake messages, incorrect output, or performance problems. Similar to a detective surveys a criminal offense scene, accumulate just as much suitable information as you are able to without having jumping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear image of what’s occurring.
Upcoming, variety hypotheses. Talk to you: What can be resulting in this habits? Have any alterations just lately been manufactured on the codebase? Has this situation occurred prior to under similar instances? The target is usually to narrow down choices and identify probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue inside of a managed surroundings. If you suspect a selected operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code queries and Enable the final results lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs often cover in the the very least expected destinations—like a lacking semicolon, an off-by-1 mistake, or perhaps a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of entirely understanding it. Short term fixes may conceal the actual difficulty, just for it to resurface later.
And finally, continue to keep notes on Everything you tried using and discovered. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and enable others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced systems.
Create Assessments
Producing checks is one of the most effective approaches to transform your debugging competencies and overall advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self esteem when earning changes to your codebase. A nicely-tested application is easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to specific features or modules. These tiny, isolated exams can swiftly reveal whether or not a specific piece of logic is working as envisioned. Any time a take a look at fails, you promptly know the place to seem, drastically lowering the time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand staying mounted.
Up coming, integrate integration assessments and conclude-to-stop tests into your workflow. These assistance be sure that a variety of elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in complex devices with several components or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable approach—serving to you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—gazing your screen for hours, making an attempt Option after solution. But Just about the most underrated debugging equipment is actually stepping away. Getting breaks will help you reset your head, cut down irritation, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-solving. A brief wander, a coffee break, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous developers report getting the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also help protect against burnout, Specially in the course of lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, nevertheless it basically contributes to a lot quicker and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing valuable in the event you make time to replicate and review what went wrong.
Begin by asking oneself a number of critical thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code opinions, or logging? The responses often reveal blind places in the workflow or understanding and help you build stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see styles—recurring troubles or widespread blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your peers is often Specially effective. Whether or not it’s through a Slack concept, a short write-up, or A fast information-sharing session, assisting others stay away from the identical issue boosts staff effectiveness and cultivates a much better Mastering tradition.
Extra importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but those who continually learn from their problems.
In the end, Every single bug you fix adds a completely new layer in your talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is huge. It helps click here make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.